Last active
June 11, 2022 10:01
-
-
Save gsusI/28dc7cce8e9264bea593470e39c8b62a to your computer and use it in GitHub Desktop.
Script to run from the browser console whist on the Wikilocs maps page. It will display options to show all trails in the map
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
function showAllMaps() { | |
let xpath = "//*[contains(@class, 'trail-list__show-on-map') and contains(text(), 'Show on map')]"; | |
let elements = document.evaluate(xpath, document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); | |
for (let i = 0; i < elements.snapshotLength; i++) { | |
if (elements.snapshotItem(i).style.display == "none") { | |
continue; | |
} | |
let title = jQuery(elements.snapshotItem(i)).closest("h3"); | |
console.log("Showing map for " + title.innerText); | |
elements.snapshotItem(i).click(); | |
} | |
} | |
function hideAllMaps() { | |
let xpath = "//*[contains(@class, 'trail-list__show-on-map') and contains(text(), 'Hide')]"; | |
let elements = document.evaluate(xpath, document, null, XPathResult.UNORDERED_NODE_SNAPSHOT_TYPE, null); | |
for (let i = 0; i < elements.snapshotLength; i++) { | |
if (elements.snapshotItem(i).style.display == "none") { | |
continue; | |
} | |
let title = jQuery(elements.snapshotItem(i)).closest("h3"); | |
console.log("Hiding map for " + title.innerText); | |
elements.snapshotItem(i).click(); | |
} | |
} | |
function showOrHideMaps() { | |
let checkbox = document.getElementById("showAllMapsCheckboxInput"); | |
if (checkbox.checked) { | |
showAllMaps(); | |
} | |
} | |
function addFloatingShowAllMapsCheckbox() { | |
var div = document.createElement("div"); | |
div.innerHTML = ` | |
<div id="showAllMapsCheckbox" | |
class="showAllMapsCheckbox draggable" | |
style=" position: fixed; top: 20px; right: 20px; z-index: 1000; width: fit-content; | |
background-color: white; | |
box-shadow: 0px 0px 10px #888888; | |
padding: 5px; | |
border-radius: 5px;"> | |
<input type="checkbox" id="showAllMapsCheckboxInput" | |
style="margin-right: 5px;" | |
onchange="updateShowAllMapsCheckboxInputValue();"> | |
Show all maps | |
</div> | |
`; | |
jQuery(document.body).append(div); | |
setTimeout(function () { | |
let showAllMapsCheckboxInput = document.getElementById("showAllMapsCheckboxInput"); | |
let showAllMapsCheckboxInputValue = localStorage.getItem("showAllMapsCheckboxInputValue"); | |
if (showAllMapsCheckboxInputValue != null) { | |
showAllMapsCheckboxInput.checked = showAllMapsCheckboxInputValue == "true"; | |
} else { | |
showAllMapsCheckboxInput.checked = false; | |
} | |
jQuery("#showAllMapsCheckbox").draggable(); | |
}, 0); | |
setInterval(showOrHideMaps, 1000); | |
} | |
function addFloatingGoThroughAllPagesCheckbox() { | |
var div = document.createElement("div"); | |
div.innerHTML = ` | |
<div id="goThroughAllPagesCheckbox"> | |
<input type="checkbox" id="goThroughAllPagesCheckboxInput" | |
style="margin-right: 5px;" | |
onchange="goThroughAllPages();"> | |
Go through all pages | |
</div> | |
`; | |
jQuery("#showAllMapsCheckbox").append(div); | |
setTimeout(function () { | |
goThroughAllPages(); | |
}, 0); | |
} | |
function addRefreshToClearResultsButton(){ | |
var div = document.createElement("div"); | |
// a tag to refresh the page keeping the search parameters | |
div.innerHTML = ` | |
<div id="refreshToClearResultsButton"> | |
<a href="javascript:void(0);" onclick="window.location.href = window.location.href;">Refresh to clear pinned trails</a> | |
</div> | |
`; | |
jQuery("#showAllMapsCheckbox").append(div); | |
} | |
function goThroughAllPages() { | |
let goThroughAllPagesCheckboxInput = document.getElementById("goThroughAllPagesCheckboxInput"); | |
if (goThroughAllPagesCheckboxInput.checked) { | |
interval = setInterval(function () { | |
let nextButton = document.getElementsByClassName("pagination__next-page")[0]; | |
if (nextButton == null) { | |
console.log("No next button found"); | |
clearInterval(interval); | |
goThroughAllPagesCheckboxInput.checked = false; | |
return; | |
} | |
console.log("Next button found"); | |
nextButton.click(); | |
}, 1500); | |
} | |
else { | |
console.log("Stopping interval: " + interval); | |
if (interval != null) { | |
clearInterval(interval); | |
} | |
} | |
} | |
function updateShowAllMapsCheckboxInputValue() { | |
let showAllMapsCheckboxInput = document.getElementById("showAllMapsCheckboxInput"); | |
localStorage.setItem("showAllMapsCheckboxInputValue", showAllMapsCheckboxInput.checked ? true : false); | |
} | |
function ensureJQueryIsLoaded() { | |
if (typeof jQuery == 'undefined') { | |
var script = document.createElement('script'); | |
script.src = 'https://ajax.googleapis.com/ajax/libs/jquery/3.3.1/jquery.min.js'; | |
script.onload = ensureJQueryIsLoaded; | |
document.getElementsByTagName('head')[0].appendChild(script); | |
return false; | |
} | |
console.log("jQuery is loaded"); | |
if (typeof jQuery.ui == 'undefined') { | |
var script = document.createElement('script'); | |
script.src = 'https://ajax.googleapis.com/ajax/libs/jqueryui/1.12.1/jquery-ui.min.js'; | |
script.onload = ensureJQueryIsLoaded; | |
document.getElementsByTagName('head')[0].appendChild(script); | |
return false; | |
} | |
console.log("JQuery UI is loaded"); | |
return true; | |
} | |
var showAllMapsCheckboxInitializationInterval = setInterval(function () { | |
if (ensureJQueryIsLoaded()) { | |
clearInterval(showAllMapsCheckboxInitializationInterval); | |
addFloatingShowAllMapsCheckbox(); | |
addFloatingGoThroughAllPagesCheckbox(); | |
addRefreshToClearResultsButton(); | |
} | |
}, 1000); |
Sign up for free
to join this conversation on GitHub.
Already have an account?
Sign in to comment
Example result:
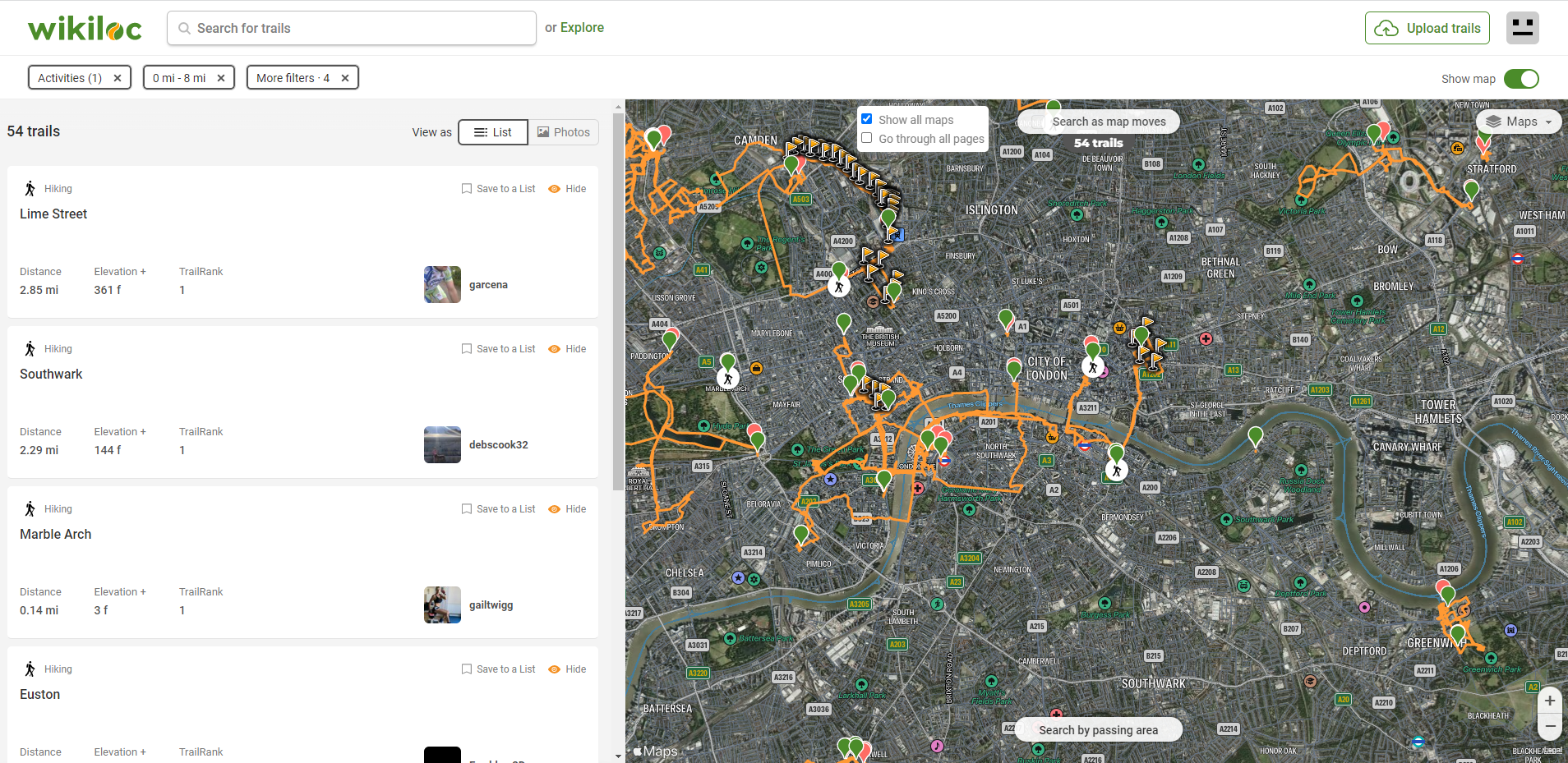